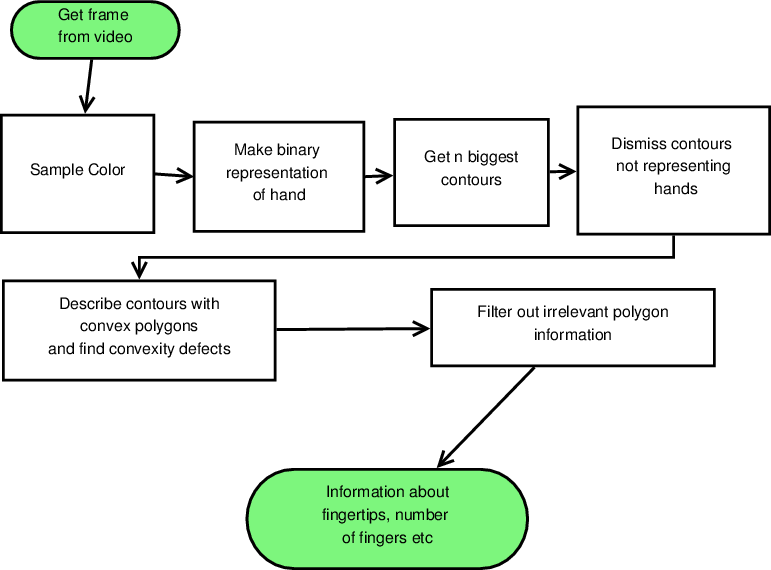
This project aims to slide images in an image gallery using handgestures. It is built with the help of opencv and c++ using a linux environment and a webcam. The source code can be found here.
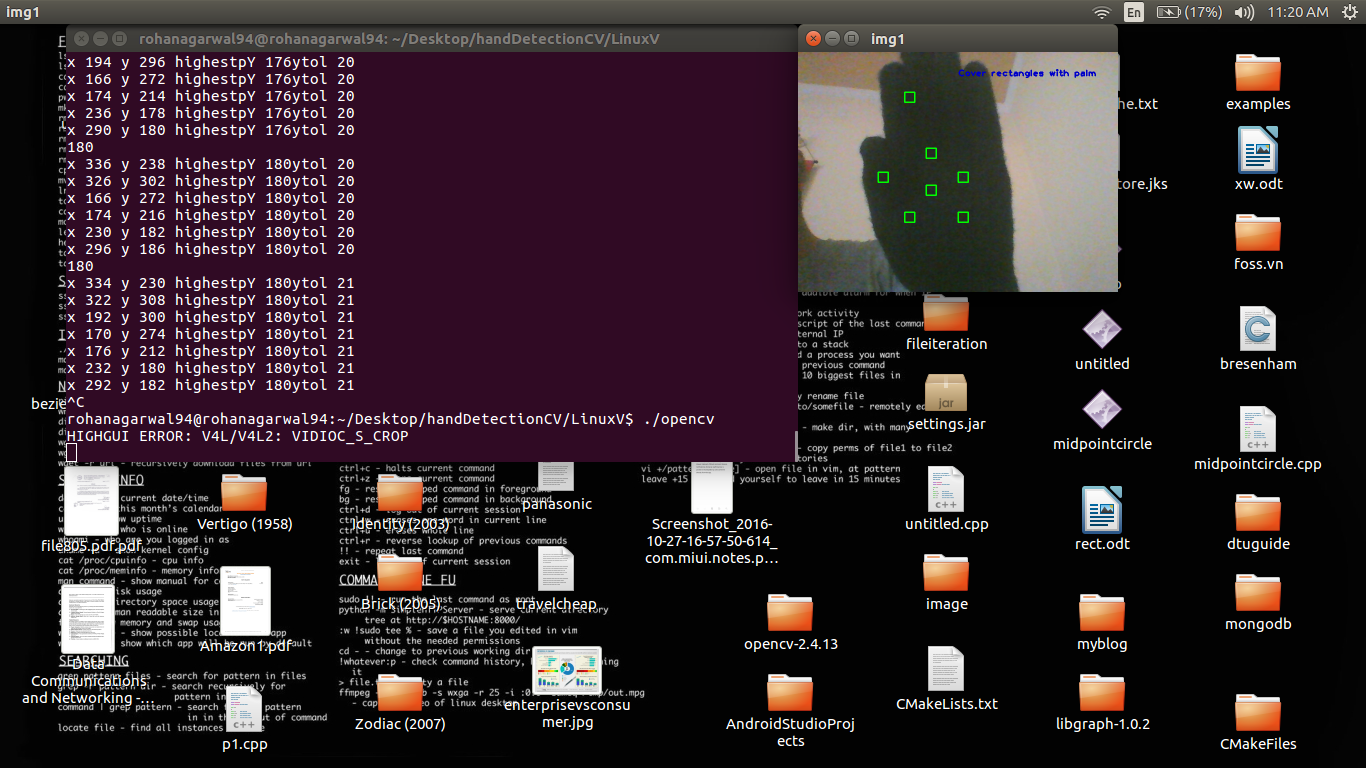
It is based on Simen Andresen’s blogpost. The hand is detected with the help of color sampling. The hand is then extracted from the background by using a threshold using the sampled color profile. Each color in the profile produces a binary image which in turn are all summed together. A nonlinear median filter is then applied to get a smooth and noise free binary representation of the hand. After that, the convexity defects are removed. The properties determining whether a convexity defect is to be dismissed is the angle between the lines going from the defect to the neighbouring convex polygon vertices.
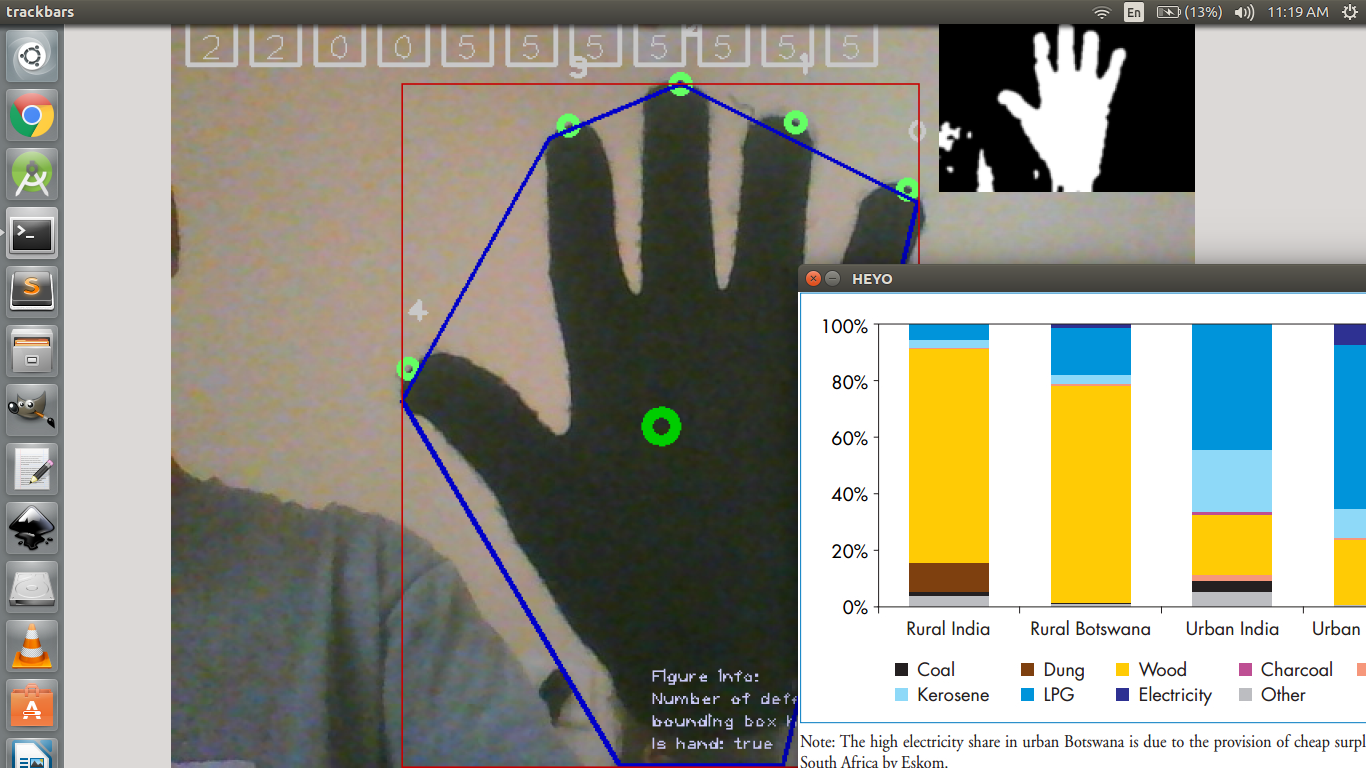
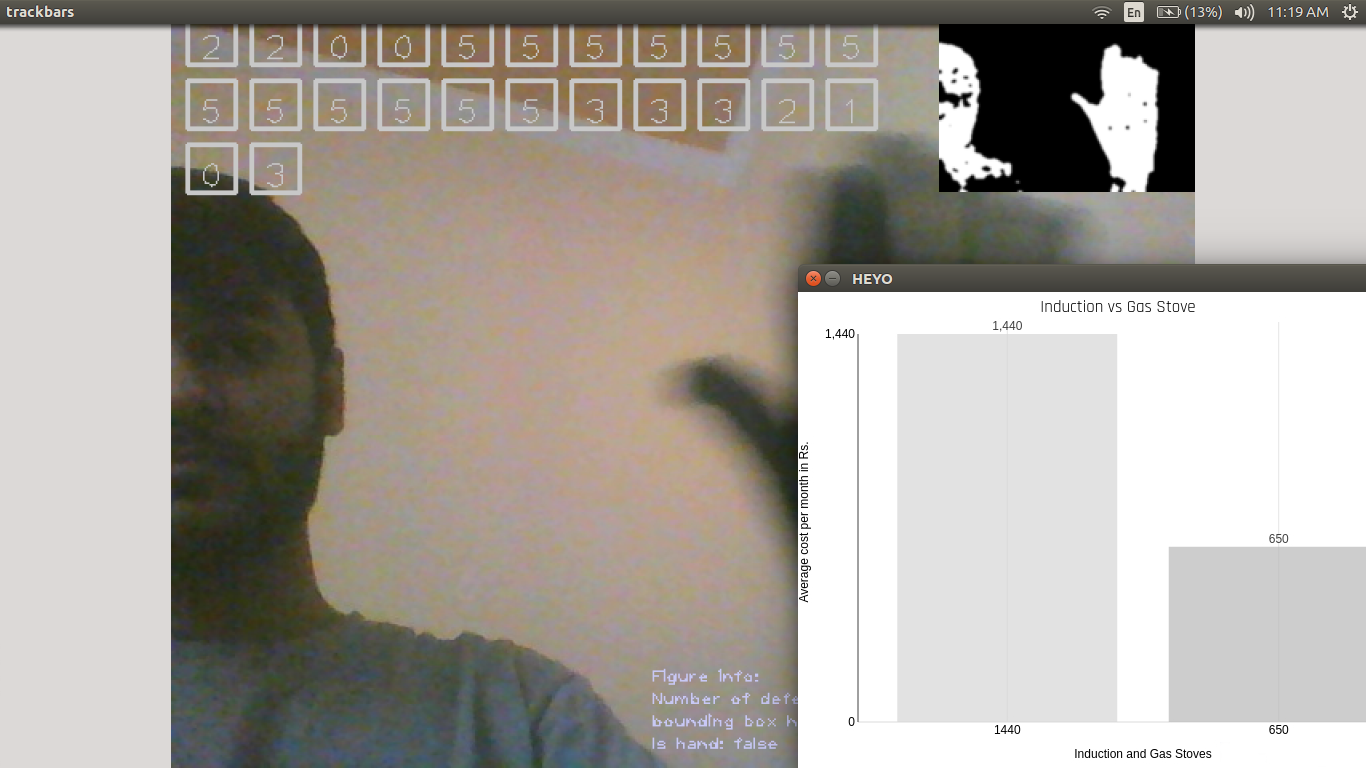
The traversal through image gallery is done by taking a folder with image suffix of jpg and incremental filenames for simplicity. Since the analysis is done frame by frame, therefore to minimise error in detecting a hand contour, a reference range is taken in the middle of the video screen. When the hand crosses a particular distance from the center of the screen(140 pixels in my case), it detects movement and hence increments/decrements the filename of the images depending upon the relative vector direction. Below is the attached code snippet which implements the sliding of the images.
1 | if(countFrame==1) |